How Builders Can Increase Their Debugging Abilities By Gustavo Woltmann
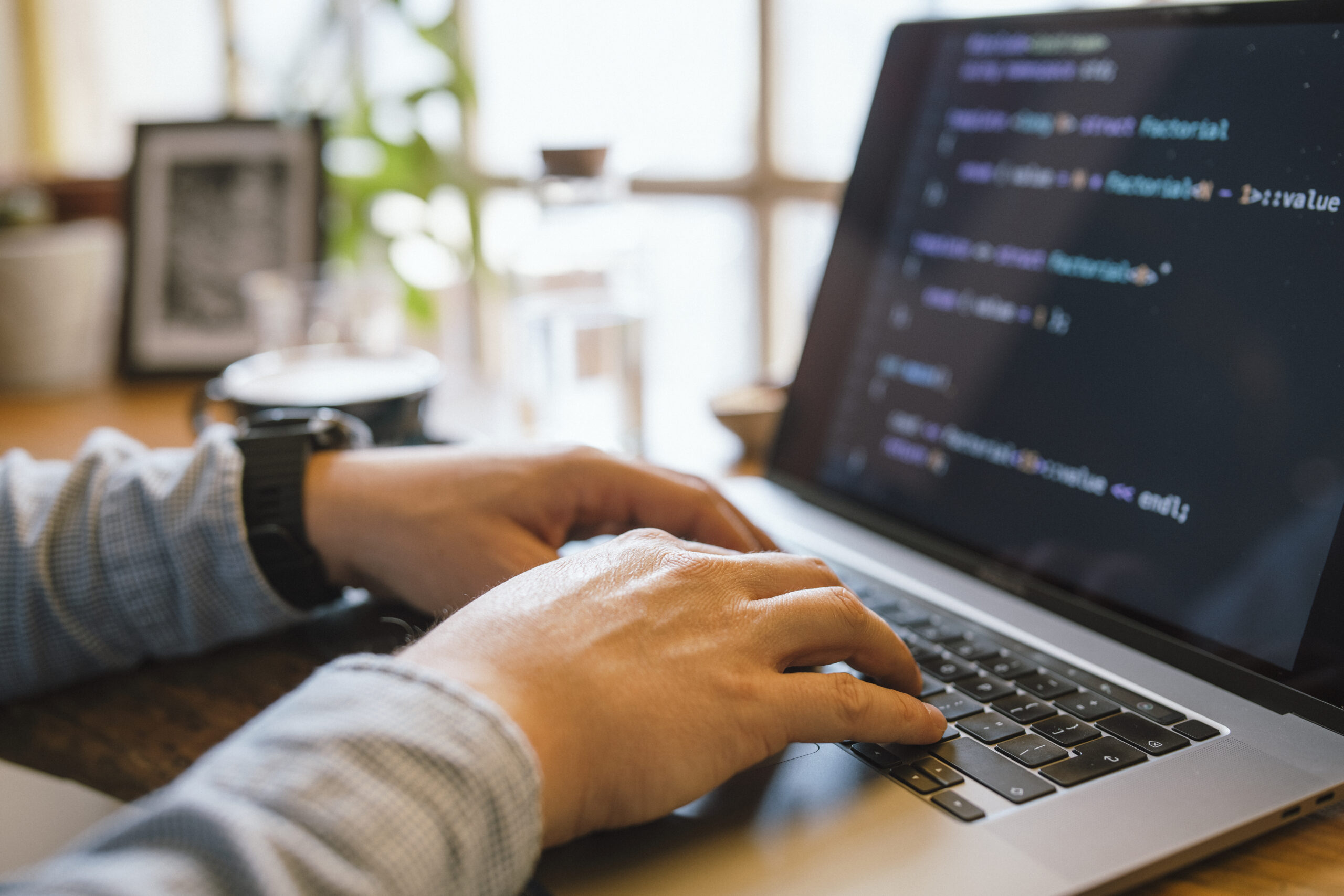
Debugging is Just about the most important — nevertheless normally overlooked — expertise in the developer’s toolkit. It's actually not pretty much correcting damaged code; it’s about understanding how and why issues go Incorrect, and Understanding to Consider methodically to unravel complications efficiently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging skills can save several hours of frustration and dramatically improve your efficiency. Here i will discuss quite a few procedures that can help builders degree up their debugging sport by me, Gustavo Woltmann.
Master Your Applications
On the list of fastest techniques developers can elevate their debugging competencies is by mastering the applications they use on a daily basis. When composing code is 1 part of enhancement, figuring out the way to interact with it correctly through execution is equally critical. Modern day development environments appear equipped with powerful debugging abilities — but a lot of developers only scratch the area of what these equipment can perform.
Just take, for instance, an Built-in Enhancement Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These applications enable you to set breakpoints, inspect the value of variables at runtime, stage as a result of code line by line, and in some cases modify code around the fly. When applied appropriately, they Permit you to notice precisely how your code behaves through execution, that is a must have for tracking down elusive bugs.
Browser developer equipment, such as Chrome DevTools, are indispensable for entrance-finish builders. They allow you to inspect the DOM, keep an eye on community requests, see real-time functionality metrics, and debug JavaScript within the browser. Mastering the console, resources, and community tabs can flip annoying UI issues into workable responsibilities.
For backend or program-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB offer you deep Command in excess of functioning processes and memory management. Mastering these applications might have a steeper Discovering curve but pays off when debugging overall performance troubles, memory leaks, or segmentation faults.
Further than your IDE or debugger, become cozy with Model Regulate techniques like Git to be familiar with code history, discover the exact second bugs have been released, and isolate problematic modifications.
In the end, mastering your equipment signifies heading outside of default configurations and shortcuts — it’s about creating an intimate knowledge of your advancement surroundings to ensure when troubles occur, you’re not shed in the dark. The greater you know your tools, the greater time you could expend solving the particular dilemma as an alternative to fumbling by way of the method.
Reproduce the trouble
Just about the most important — and sometimes neglected — measures in successful debugging is reproducing the issue. Prior to leaping into the code or making guesses, builders need to have to create a consistent environment or state of affairs where by the bug reliably seems. Without having reproducibility, fixing a bug results in being a match of likelihood, frequently leading to wasted time and fragile code improvements.
Step one in reproducing a problem is accumulating as much context as possible. Ask issues like: What actions triggered The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have, the much easier it gets to be to isolate the precise situations below which the bug takes place.
After you’ve gathered adequate information, try and recreate the issue in your local natural environment. This could signify inputting exactly the same facts, simulating equivalent person interactions, or mimicking method states. If The difficulty appears intermittently, take into consideration composing automatic tests that replicate the sting instances or state transitions included. These checks not just enable expose the problem but in addition reduce regressions Sooner or later.
In some cases, the issue could possibly be ecosystem-particular — it would materialize only on specific functioning methods, browsers, or beneath unique configurations. Using equipment like Digital equipment, containerization (e.g., Docker), or cross-browser tests platforms can be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t simply a step — it’s a attitude. It involves tolerance, observation, as well as a methodical tactic. But as you can consistently recreate the bug, you're presently midway to repairing it. With a reproducible scenario, You should use your debugging resources far more properly, take a look at probable fixes properly, and talk far more Plainly with the workforce or buyers. It turns an summary criticism into a concrete obstacle — Which’s wherever builders prosper.
Read through and Fully grasp the Mistake Messages
Mistake messages in many cases are the most beneficial clues a developer has when a little something goes Completely wrong. Rather then looking at them as discouraging interruptions, builders should really study to deal with error messages as immediate communications with the technique. They usually tell you what exactly occurred, exactly where it transpired, and from time to time even why it occurred — if you know how to interpret them.
Get started by looking through the concept very carefully and in whole. Several developers, particularly when below time pressure, look at the primary line and instantly start building assumptions. But deeper during the error stack or logs may lie the legitimate root lead to. Don’t just copy and paste mistake messages into engines like google — read and fully grasp them very first.
Crack the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it issue to a particular file and line quantity? What module or purpose induced it? These issues can guidebook your investigation and issue you toward the dependable code.
It’s also helpful to understand the terminology on the programming language or framework you’re using. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially quicken your debugging course of action.
Some errors are obscure or generic, As well as in Those people circumstances, it’s important to look at the context wherein the error occurred. Check out similar log entries, input values, and recent adjustments from the codebase.
Don’t overlook compiler or linter warnings both. These generally precede larger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Discovering to interpret them correctly turns chaos into clarity, assisting you pinpoint concerns more rapidly, lower debugging time, and turn into a extra efficient and confident developer.
Use Logging Correctly
Logging is Among the most impressive applications in a developer’s debugging toolkit. When used successfully, it provides genuine-time insights into how an application behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or action from the code line by line.
A fantastic logging tactic starts with knowing what to log and at what amount. Popular logging concentrations include things like DEBUG, Details, Alert, ERROR, and FATAL. Use DEBUG for in depth diagnostic details throughout improvement, INFO for general situations (like prosperous start off-ups), WARN for likely concerns that don’t break the applying, Mistake for real problems, and Lethal if the technique can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. Far too much logging can obscure critical messages and slow down your procedure. Center on crucial events, state improvements, input/output values, and important determination points in the code.
Format your log messages Evidently and persistently. Incorporate context, like timestamps, ask for IDs, and function names, so it’s much easier to trace problems in dispersed techniques or multi-threaded environments. Structured logging (e.g., JSON logs) will make it even simpler to parse and filter logs programmatically.
In the course of debugging, logs Permit you to monitor how variables evolve, what disorders are satisfied, and what branches of logic are executed—all without the need of halting the program. They’re Primarily useful in production environments the place stepping by means of code isn’t probable.
Furthermore, use logging frameworks and instruments (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. With a perfectly-believed-out logging technique, you can reduce the time it will require to identify problems, achieve further visibility into your applications, and Enhance the In general maintainability and reliability of the code.
Assume Similar to a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To proficiently detect and repair bugs, developers have to tactic the procedure like a detective solving a thriller. This frame of mind assists break down intricate difficulties into workable pieces and follow clues logically to uncover the root trigger.
Commence by collecting proof. Think about the symptoms of the issue: error messages, incorrect output, or overall performance concerns. Similar to a detective surveys a criminal offense scene, gather as much suitable facts as you may without the need of leaping to conclusions. Use logs, take a look at scenarios, and consumer studies to piece collectively a clear photo of what’s taking place.
Up coming, type hypotheses. Inquire your self: What might be causing this actions? Have any changes recently been produced to the codebase? Has this issue happened ahead of beneath comparable circumstances? The intention will be to slim down prospects and recognize possible culprits.
Then, exam your theories systematically. Endeavor to recreate the challenge within a controlled natural environment. In case you suspect a specific functionality or element, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the results guide you closer to the reality.
Pay out close notice to modest particulars. Bugs normally conceal in the minimum expected spots—like a lacking semicolon, an off-by-just one error, or maybe a race problem. Be complete and affected person, resisting the urge to patch The difficulty without having here absolutely knowing it. Non permanent fixes could hide the true trouble, only for it to resurface afterwards.
Lastly, hold notes on what you experimented with and acquired. Just as detectives log their investigations, documenting your debugging approach can help you save time for long run issues and aid Many others comprehend your reasoning.
By contemplating similar to a detective, developers can sharpen their analytical expertise, tactic problems methodically, and grow to be more practical at uncovering hidden concerns in advanced units.
Create Exams
Composing assessments is one of the most effective approaches to transform your debugging abilities and All round growth performance. Checks don't just help catch bugs early but also serve as a safety net that provides you self esteem when building variations to your codebase. A nicely-tested application is easier to debug because it enables you to pinpoint precisely exactly where and when a challenge happens.
Begin with unit exams, which give attention to personal features or modules. These tiny, isolated exams can rapidly reveal whether a specific piece of logic is working as expected. Any time a exam fails, you promptly know wherever to seem, drastically minimizing time invested debugging. Device tests are Primarily handy for catching regression bugs—troubles that reappear right after Formerly being preset.
Following, integrate integration tests and conclusion-to-conclude assessments into your workflow. These assist ensure that many areas of your application do the job jointly easily. They’re particularly handy for catching bugs that take place in complex devices with many elements or services interacting. If a thing breaks, your tests can show you which Portion of the pipeline unsuccessful and beneath what conditions.
Producing tests also forces you to definitely think critically about your code. To check a feature adequately, you'll need to be aware of its inputs, anticipated outputs, and edge scenarios. This degree of knowledge In a natural way leads to higher code composition and fewer bugs.
When debugging a difficulty, creating a failing take a look at that reproduces the bug may be a strong starting point. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your take a look at pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, producing checks turns debugging from the irritating guessing match right into a structured and predictable system—supporting you capture extra bugs, quicker and even more reliably.
Acquire Breaks
When debugging a tough issue, it’s simple to become immersed in the trouble—observing your display screen for several hours, seeking Remedy soon after Alternative. But one of the most underrated debugging resources is just stepping away. Using breaks aids you reset your brain, lessen stress, and sometimes see The problem from a new viewpoint.
When you are way too near to the code for way too prolonged, cognitive tiredness sets in. You could possibly start off overlooking clear problems or misreading code which you wrote just hours earlier. In this point out, your Mind becomes less economical at trouble-resolving. A short walk, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your emphasis. A lot of builders report locating the root of a dilemma when they've taken time for you to disconnect, letting their subconscious do the job from the qualifications.
Breaks also assist prevent burnout, Primarily for the duration of for a longer time debugging sessions. Sitting before a display, mentally stuck, is not simply unproductive but in addition draining. Stepping away means that you can return with renewed Vitality along with a clearer mentality. You could possibly all of a sudden see a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In case you’re stuck, a superb rule of thumb will be to set a timer—debug actively for forty five–60 minutes, then take a five–10 moment break. Use that point to maneuver close to, extend, or do one thing unrelated to code. It may come to feel counterintuitive, Particularly underneath tight deadlines, nonetheless it actually contributes to faster and simpler debugging Ultimately.
In brief, getting breaks is not a sign of weak point—it’s a sensible method. It gives your brain Room to breathe, increases your perspective, and will help you steer clear of the tunnel vision that often blocks your development. Debugging is usually a mental puzzle, and rest is a component of resolving it.
Learn From Each and every Bug
Just about every bug you come across is a lot more than simply a temporary setback—It is a chance to improve as a developer. Regardless of whether it’s a syntax mistake, a logic flaw, or maybe a deep architectural difficulty, each one can teach you one thing worthwhile for those who take the time to reflect and assess what went Completely wrong.
Start by asking your self several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught before with better practices like device tests, code reviews, or logging? The responses frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding routines moving ahead.
Documenting bugs will also be a wonderful pattern. Retain a developer journal or retain a log in which you Notice down bugs you’ve encountered, how you solved them, and what you discovered. As time passes, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively steer clear of.
In team environments, sharing Anything you've uncovered from a bug with your friends might be Specifically potent. Whether it’s by way of a Slack concept, a short generate-up, or A fast information-sharing session, assisting Many others stay away from the exact same difficulty boosts staff efficiency and cultivates a much better Understanding culture.
Additional importantly, viewing bugs as lessons shifts your mentality from disappointment to curiosity. Instead of dreading bugs, you’ll start out appreciating them as crucial elements of your enhancement journey. All things considered, some of the finest developers are certainly not the ones who publish perfect code, but individuals who continuously understand from their mistakes.
In the long run, each bug you resolve provides a brand new layer on your skill set. So next time you squash a bug, take a minute to replicate—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the payoff is huge. It helps make you a far more economical, confident, and capable developer. The subsequent time you might be knee-deep within a mysterious bug, recall: debugging isn’t a chore — it’s a possibility to become much better at Whatever you do.